If you include sqrt function lives in std namespace. So declare using namespace std; or (better) use std::sqrt(.) or declare using std::sqrt. Probably, that's all your troubles now. Jun 25, 2003 You will have to declare variables to input the data. Luckily, declaring variables in C is very easy. This simple program gets the user's age as input and displays it back to the screen. Here is the source code: #include using namespace std; int main int age; cout C Program'. C Formatting Output Tutorial - Formatting output is important in the development of output screen, which can be easily read and understood. C offers the programmer several input/output manipulators.
A char variable in C++ is designed to hold an ASCII character, an int an integer number, and a double a floating-point number. Similarly, a pointer variable is designed to hold a memory address. You declare a pointer variable by adding an asterisk (*) to the end of the type of the object that the pointer points at, as in the following example:
A pointer variable that has not otherwise been initialized contains an unknown value. Using the ampersand (&) operator, you can initialize a pointer variable with the address of a variable of the same type:
In this snippet, the variable cSomeChar has some address. For argument’s sake, say that C++ assigned it the address 0x1000. (C++ also initialized that location with the character ‘a’.) The variable pChar also has a location of its own, perhaps 0x1004. The value of the expression &cSomeChar is 0x1000, and its type is char* (read “pointer to char”).
Experience the Precision Tune Difference Precision Tune Auto Care celebrates 40 years of taking care of cars and the people who ride in them, providing car owners with a one-stop shop for factory scheduled. About Precision Tune Auto Care Precision Tune Auto Care began as a tune-up shop in Beaumont, Texas in 1977. Today, there are more than 250 locations throughout the United States. The company services. Where synthetic oil is required, Precision Tune Auto Care carries the necessary oils for your vehicle. Synthetic Motor Oil is manufactured for better wear and flow characteristics as well as superior. Precision auto tune synthetic oil change coupons near me. To get great deals from Precision Tune Auto Care, use any of these 5 coupons. This list is updated every day in April to make sure you never pay more than you have to at your local store.
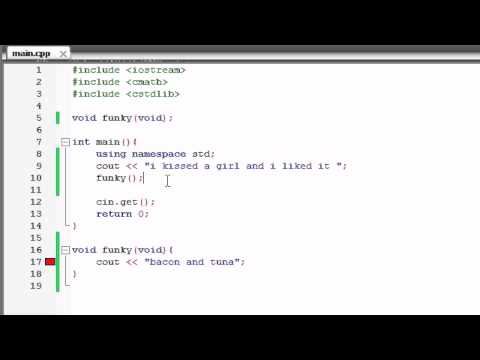
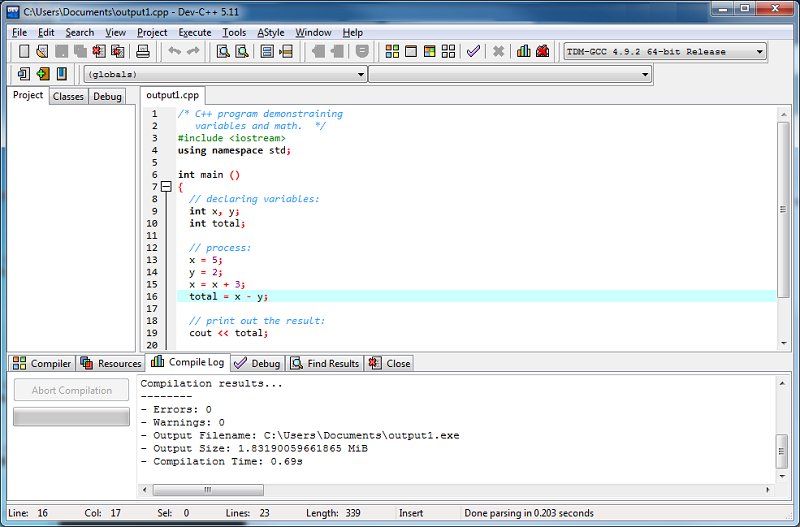
So the assignment on the third line of the snippet example stores the value 0x1000 in the variable pChar.
Take a minute to really understand the relationship between the figure and the three lines of C++ code in the snippet. The first declaration says, “go out and find a 1-byte location in memory, assign it the name cSomeChar, and initialize it to ‘a’.” In this example, C++ picked the location 0x1000.
The next line says, “go out and find a location large enough to hold the address of a char variable and assign it the name pChar.” In this example, C++ assigned pChar to the location 0x1004.
The third line says, “assign the address of cSomeChar (0x1000) to the variable pChar.” The figure represents the state of the program after these three statements.
“So what?” you say. Here comes the really cool part, as demonstrated in the following expression:
This line says, “store a ‘b’ at the char location pointed at by pChar.” This is demonstrated in the following figure. To execute this expression, C++ first retrieves the value stored in pChar (that would be 0x1000). It then stores the character ‘b’ at that location.
How To Declare Cout In Dev C Online
The * when used as a binary operator means “multiply”; when used as a unary operator, * means “find the thing pointed at by.” Similarly & has a meaning as a binary operator, but as a unary operator, it means “take the address of.”
Header File For Cout In Dev C++
So what’s so exciting about that? After all, you could achieve the same effect by simply assigning a ‘b’ to cSomeChar directly:
Why go through the intermediate step of retrieving its address in memory? Because there are several problems that can be solved only with pointers.
- C++ Basics
- C++ Object Oriented
- C++ Advanced
- C++ Useful Resources
- Selected Reading
C++ provides following two types of string representations −
- The C-style character string.
- The string class type introduced with Standard C++.
The C-Style Character String
The C-style character string originated within the C language and continues to be supported within C++. This string is actually a one-dimensional array of characters which is terminated by a null character '0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.

How To Declare Cout In Dev C File
The following declaration and initialization create a string consisting of the word 'Hello'. To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word 'Hello.'
If you follow the rule of array initialization, then you can write the above statement as follows −
Following is the memory presentation of above defined string in C/C++ −
Actually, you do not place the null character at the end of a string constant. The C++ compiler automatically places the '0' at the end of the string when it initializes the array. Let us try to print above-mentioned string −
How To Declare Cout In Dev C 4
When the above code is compiled and executed, it produces the following result −
C++ supports a wide range of functions that manipulate null-terminated strings −
Just download and start playing it. Free download for samsung mobile. Cooking Simulator PC Game free. download full Version full and complete game. Direct links to download this game is given below.
Sr.No | Function & Purpose |
---|---|
1 | strcpy(s1, s2); Copies string s2 into string s1. |
2 | strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | strlen(s1); Returns the length of string s1. |
4 | strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
Following example makes use of few of the above-mentioned functions −
When the above code is compiled and executed, it produces result something as follows −
The String Class in C++
The standard C++ library provides a string class type that supports all the operations mentioned above, additionally much more functionality. Let us check the following example − Free little snitch alternatives.
How To Declare Cout In Dev C Pdf
When the above code is compiled and executed, it produces result something as follows −